GitHub - fabulous-dev/Fabulous: Declarative UI framework for cross-platform mobile & desktop apps, using MVU and F# functional programmingDeclarative UI framework for cross-platform mobile & desktop apps, using MVU and F# functional programming - fabulous-dev/Fabulous
Visit Site
GitHub - fabulous-dev/Fabulous: Declarative UI framework for cross-platform mobile & desktop apps, using MVU and F# functional programming
Fabulous is a modern declarative UI framework for crafting cross-platform mobile and desktop apps in .NET.
It aims to bring you a great development experience and confidence in your code by combining an expressive UI syntax, the simple & robust Model-View-Update (MVU) architecture, and functional programming.
Documentation
The full documentation for Fabulous can be found at docs.fabulous.dev.
Other useful links:
Additionally, we have the Fabulous Discord server where you can ask any of your Fabulous related questions.
About Fabulous
We believe declarative UI, functional programming, and the MVU state management are a perfect fit for app development.
Fabulous will help you create mobile and desktop apps quickly and with confidence thanks to declarative UI and the MVU architecture, all in one single language: F# - a functional programing language.
Fabulous also aims to be performant by having low memory consumption and efficient view diffing mechanisms.
Note that Fabulous itself does not provide any UI rendering. You'll need to combine it with another framework like:
Declarative UI
Typical UI development can be a nightmare if not done properly.
It is generally created in one place, then mutated here and there based on the need and what the user is doing. Related UI pieces end up in several places, making it hard to mentally think of all the possibilities; until the inevitable race condition or bug due to an unintended user flow.
Fabulous makes it easier to reason about UI thanks to its declarative UI inspired by SwiftUI.
The UI of a component is defined in a single place and Fabulous will call it everytime the state of that component is changed.
You don't need to think about how to mutate the UI, Fabulous will handle it for you to always match the latest UI you need.
/// A simple Counter app made with Fabulous.MauiControls
type Model =
{ Count: int }
type Msg =
| Increment
| Decrement
let view model =
Application(
ContentPage(
"Counter app",
VStack(spacing = 16.) {
Image(Aspect.AspectFit, "fabulous.png")
Label($"Count is {model.Count}")
Button("Increment", Increment)
Button("Decrement", Decrement)
}
)
)
MVU architecture
MVU makes every state and transition between those states explicit.
You don't need to worry about unintended actions that could lead to an invalid state which would crash the app.
Instead, you can very easily model the state of your app or component and transitions between them using F# records and discriminated unions types.
When starting, Fabulous will initialize the state. Then, when messages are being dispatched, Fabulous will let you transition from one state to the other given a specific message.
If several messages are received at the same time, Fabulous will queue them to let you update the state properly.
let init () =
{ Count = 0 }
let update msg model =
match msg with
| Increment -> { model with Count = model.Count + 1 }
| Decrement -> { model with Count = model.Count - 1 }
And finally, given the functional nature of MVU, it is extremely simple to unit test each and every possible state of your application.
[<Test>]
let ``When clicking the Increment button, increment the count by one``() =
let previousState = { Count = 10 }
let expectedState = { Count = 11 }
let actualState = App.update Increment previousState
actualState |> should equal expectedState
Powered by .NET
.NET is a very mature and broad framework by Microsoft. It can run on any device and platform, is very efficient, and has a vast ecosystem of open-source and licensed libraries, plugins, and other frameworks.
You will be able to benefit from the .NET ecosystem by using 3rd party packages directly in your Fabulous application.
Supporting this project
The simplest way to show us your support is by giving the project a star.
You can also support us by becoming our sponsor on the GitHub Sponsors program.
This is a fantastic way to support all the efforts going into making Fabulous the best declarative UI framework for dotnet.
If you need support see Commercial Support section below.
Contributing
Have you found a bug or have a suggestion of how to enhance Fabulous? Open an issue and we will take a look at it as soon as possible.
Do you want to contribute with a PR? PRs are always welcome, just make sure to create it from the correct branch (main) and follow the Contributor Guide.
For bigger changes, or if in doubt, make sure to talk about your contribution to the team. Either via an issue, GitHub discussion, or reach out to the team either using the Discord server.
Commercial support
If you would like us to provide you with:
- training and workshops,
- support services,
- and consulting services.
Feel free to contact us: [email protected]
Star History
More Resourcesto explore the angular.
mail [email protected] to add your project or resources here ๐ฅ.
- 1compiler/hints at master ยท elm/compiler
https://github.com/elm/compiler/tree/master/hints
Compiler for Elm, a functional language for reliable webapps. - elm/compiler
- 2Start an Elm SPA ready to the real world
https://github.com/rogeriochaves/spades
Start an Elm SPA ready to the real world. Contribute to rogeriochaves/spades development by creating an account on GitHub.
- 3Kanban board built with Elm
https://github.com/huytd/kanelm
Kanban board built with Elm. Contribute to huytd/kanelm development by creating an account on GitHub.
- 4`elm make` in watch mode. Fast and reliable.
https://github.com/lydell/elm-watch
`elm make` in watch mode. Fast and reliable. Contribute to lydell/elm-watch development by creating an account on GitHub.
- 5๐พ JS type systems interportability
https://github.com/stereobooster/type-o-rama
๐พ JS type systems interportability. Contribute to stereobooster/type-o-rama development by creating an account on GitHub.
- 6The secure, private journal.
https://github.com/tarbh-engineering/journal
The secure, private journal. Contribute to tarbh-engineering/journal development by creating an account on GitHub.
- 7Yeoman generator for elm-mdl in pure elm
https://github.com/ashellwig/generator-elm-mdl
Yeoman generator for elm-mdl in pure elm. Contribute to ashellwig/generator-elm-mdl development by creating an account on GitHub.
- 8Hacker News as a PWA built with Elm
https://github.com/elmariofredo/elm-hn-pwa
Hacker News as a PWA built with Elm. Contribute to elmariofredo/elm-hn-pwa development by creating an account on GitHub.
- 9๐พ Generate a new Elm project from the command line (Elm 0.16+)
https://github.com/simonewebdesign/elm-new
๐พ Generate a new Elm project from the command line (Elm 0.16+) - simonewebdesign/elm-new
- 10A tool that allows you to analyse your Elm code, identify deficiencies and apply best practices.
https://github.com/stil4m/elm-analyse
A tool that allows you to analyse your Elm code, identify deficiencies and apply best practices. - stil4m/elm-analyse
- 11Bringing the wonders of Elm to the iOS platform
https://github.com/pzp1997/elm-ios
Bringing the wonders of Elm to the iOS platform. Contribute to pzp1997/elm-ios development by creating an account on GitHub.
- 12Haskell: Derive Elm types from Haskell types
https://github.com/agrafix/elm-bridge
Haskell: Derive Elm types from Haskell types. Contribute to agrafix/elm-bridge development by creating an account on GitHub.
- 13articles/switching_from_imperative_to_functional_programming_with_games_in_Elm.md at master ยท Dobiasd/articles
https://github.com/Dobiasd/articles/blob/master/switching_from_imperative_to_functional_programming_with_games_in_Elm.md
thoughts on programming. Contribute to Dobiasd/articles development by creating an account on GitHub.
- 14The TodoMVC app written in Elm, nice example for beginners.
https://github.com/evancz/elm-todomvc
The TodoMVC app written in Elm, nice example for beginners. - evancz/elm-todomvc
- 15A tool for building interactive fiction style stories in Elm.
https://github.com/jschomay/elm-narrative-engine
A tool for building interactive fiction style stories in Elm. - jschomay/elm-narrative-engine
- 16Grunt plugin that compiles Elm files to JavaScript.
https://github.com/rtfeldman/grunt-elm
Grunt plugin that compiles Elm files to JavaScript. - rtfeldman/grunt-elm
- 17Run Elm code from the command line
https://github.com/jfairbank/run-elm
Run Elm code from the command line. Contribute to jfairbank/run-elm development by creating an account on GitHub.
- 18Initialise scaffolding for a new Elm project
https://github.com/JustusAdam/elm-init
Initialise scaffolding for a new Elm project. Contribute to JustusAdam/elm-init development by creating an account on GitHub.
- 19๐ Create Elm apps with zero configuration
https://github.com/halfzebra/create-elm-app
๐ Create Elm apps with zero configuration. Contribute to halfzebra/create-elm-app development by creating an account on GitHub.
- 20:book: Practical examples in Elm
https://github.com/halfzebra/elm-examples
:book: Practical examples in Elm. Contribute to halfzebra/elm-examples development by creating an account on GitHub.
- 21:school_satchel: Elm kit is web application boilerplate kit for development. This kit build on Brunch, Node, Sass, Elm-lang. It helps you to start development more productive following best practices.
https://github.com/khusnetdinov/elmkit
:school_satchel: Elm kit is web application boilerplate kit for development. This kit build on Brunch, Node, Sass, Elm-lang. It helps you to start development more productive following best practic...
- 22All Elm Games (hopefully)
https://github.com/rofrol/elm-games
All Elm Games (hopefully). Contribute to rofrol/elm-games development by creating an account on GitHub.
- 23Experiment to compile something Elm-ish to Wasm
https://github.com/Chadtech/elmish-wasm
Experiment to compile something Elm-ish to Wasm. Contribute to Chadtech/elmish-wasm development by creating an account on GitHub.
- 24A set of koans for learning Elm
https://github.com/robertjlooby/elm-koans
A set of koans for learning Elm. Contribute to robertjlooby/elm-koans development by creating an account on GitHub.
- 25Rework Vim install instructions ยท Issue #610 ยท avh4/elm-format
https://github.com/avh4/elm-format/issues/610
The current recommendation is to use elmcast/elm-vim. As of today, I'd not recommend using this plugin, because Elm 0.19 is still not supported for some things: ElmCast/elm-vim#182 and other issues...
- 26How to create modular Elm code that scales nicely with your app
https://github.com/evancz/elm-architecture-tutorial
How to create modular Elm code that scales nicely with your app - evancz/elm-architecture-tutorial
- 27Describe the behavior of Elm programs
https://github.com/brian-watkins/elm-spec
Describe the behavior of Elm programs. Contribute to brian-watkins/elm-spec development by creating an account on GitHub.
- 28Install, upgrade and uninstall Elm dependencies
https://github.com/zwilias/elm-json
Install, upgrade and uninstall Elm dependencies. Contribute to zwilias/elm-json development by creating an account on GitHub.
- 29Literate Visualization: Theory, software and examples
https://github.com/gicentre/litvis
Literate Visualization: Theory, software and examples - gicentre/litvis
- 30A collection of tips for people using Elm from a web-dev background
https://github.com/eeue56/elm-for-web-developers
A collection of tips for people using Elm from a web-dev background - eeue56/elm-for-web-developers
- 31An overview of Elm syntax and features
https://github.com/izdi/elm-cheat-sheet
An overview of Elm syntax and features. Contribute to izdi/elm-cheat-sheet development by creating an account on GitHub.
- 32An example Elm single page application
https://github.com/sporto/elm-example-app
An example Elm single page application. Contribute to sporto/elm-example-app development by creating an account on GitHub.
- 33The "Hello world" of Elm + Web Components.
https://github.com/ohanhi/elm-ement
The "Hello world" of Elm + Web Components. Contribute to ohanhi/elm-ement development by creating an account on GitHub.
- 34An example of the ELM architecture on Android using Kotlin with Anko
https://github.com/glung/elm-architecture-android
An example of the ELM architecture on Android using Kotlin with Anko - glung/elm-architecture-android
- 35Command Line Utility for creating an Elm boilerplate project easy to run, build and get deployed
https://github.com/GioPat/elm-boil
Command Line Utility for creating an Elm boilerplate project easy to run, build and get deployed - GioPat/elm-boil
- 36eeue56/haskell-to-elm
https://github.com/eeue56/haskell-to-elm
Contribute to eeue56/haskell-to-elm development by creating an account on GitHub.
- 37The Pragmatic Studio
https://pragmaticstudio.com/elm
Premium video courses for software developers. Real apps. Real code. Really good stuff!
- 38A simple Makefile able to create a new Elm app
https://github.com/guillaumearm/elm-boilerplate
A simple Makefile able to create a new Elm app. Contribute to guillaumearm/elm-boilerplate development by creating an account on GitHub.
- 39A plugin for Vite enables you to compile an Elm application/document/element
https://github.com/hmsk/vite-plugin-elm
A plugin for Vite enables you to compile an Elm application/document/element - hmsk/vite-plugin-elm
- 40Elm snippets for Atom :kissing_heart:
https://github.com/chiefGui/atom-elm-snippets
Elm snippets for Atom :kissing_heart:. Contribute to chiefGui/atom-elm-snippets development by creating an account on GitHub.
- 41Chrome extension in elm that shows the latest currency bitcoins from Brazilian exchanges
https://github.com/jouderianjr/bitcoin-br-chrome-extension
Chrome extension in elm that shows the latest currency bitcoins from Brazilian exchanges - jouderianjr/bitcoin-br-chrome-extension
- 42Elm offline documentation previewer
https://github.com/dmy/elm-doc-preview
Elm offline documentation previewer. Contribute to dmy/elm-doc-preview development by creating an account on GitHub.
- 43Elm plugin for Vim
https://github.com/ElmCast/elm-vim
Elm plugin for Vim. Contribute to ElmCast/elm-vim development by creating an account on GitHub.
- 44:rainbow: discover the beautiful programming language that makes front-end web apps a joy to build and maintain!
https://github.com/dwyl/learn-elm
:rainbow: discover the beautiful programming language that makes front-end web apps a joy to build and maintain! - dwyl/learn-elm
- 45Conway's Game of Life
https://github.com/pecheneg2015/elm-conway-life
Conway's Game of Life. Contribute to pecheneg2015/elm-conway-life development by creating an account on GitHub.
- 46โกโA flexible dev server for Elm. Live reload included.
https://github.com/wking-io/elm-live
โกโA flexible dev server for Elm. Live reload included. - wking-io/elm-live
- 47mdgriffith/elm-codegen
https://github.com/mdgriffith/elm-codegen
Contribute to mdgriffith/elm-codegen development by creating an account on GitHub.
- 48avh4/elm-program-test at 3.0.0
https://github.com/avh4/elm-program-test/tree/3.0.0
Test Elm programs. Contribute to avh4/elm-program-test development by creating an account on GitHub.
- 49Command line tool to share Elm libraries
https://github.com/elm-lang/elm-package
Command line tool to share Elm libraries. Contribute to elm-lang/elm-package development by creating an account on GitHub.
- 50An app built with Elm to explore new artists using the Spotify api.
https://github.com/FidelisClayton/elm-spotify-mapper
An app built with Elm to explore new artists using the Spotify api. - FidelisClayton/elm-spotify-mapper
- 51Example showing hot module reloading for Elm 0.19 and Webpack
https://github.com/klazuka/example-elm-hot-webpack
Example showing hot module reloading for Elm 0.19 and Webpack - klazuka/example-elm-hot-webpack
- 52Hey there!
https://www.brianthicks.com
Iโm Brian Hicks. I work with and write about Elm, organize elm-conf, and run the State of Elm โฆ
- 53Generate TypeScript declaration files for your elm ports!
https://github.com/dillonkearns/elm-typescript-interop
Generate TypeScript declaration files for your elm ports! - dillonkearns/elm-typescript-interop
- 54Modular, heavily-documented Elm todo app with a json rest api
https://github.com/andrewsuzuki/elm-todo-rest-api
Modular, heavily-documented Elm todo app with a json rest api - andrewsuzuki/elm-todo-rest-api
- 55Webpack loader for the Elm programming language.
https://github.com/elm-community/elm-webpack-loader
Webpack loader for the Elm programming language. Contribute to elm-community/elm-webpack-loader development by creating an account on GitHub.
- 56Write CLI-scripts in Elm
https://github.com/albertdahlin/elm-posix
Write CLI-scripts in Elm. Contribute to albertdahlin/elm-posix development by creating an account on GitHub.
- 57Elm-like abstractions for F# apps
https://github.com/elmish/elmish
Elm-like abstractions for F# apps. Contribute to elmish/elmish development by creating an account on GitHub.
- 58TodoMVC+Firebase in Elm+ElmFire
https://github.com/ThomasWeiser/todomvc-elmfire
TodoMVC+Firebase in Elm+ElmFire. Contribute to ThomasWeiser/todomvc-elmfire development by creating an account on GitHub.
- 59Write unit and fuzz tests for Elm code.
https://github.com/elm-explorations/test
Write unit and fuzz tests for Elm code. Contribute to elm-explorations/test development by creating an account on GitHub.
- 60The Space App
https://github.com/thematters/thespace-app
The Space App. Contribute to thematters/thespace-app development by creating an account on GitHub.
- 61Elm in Action
https://www.manning.com/books/elm-in-action
Elm is more than just a cutting-edge programming language, itโs a chance to upgrade the way you think about building web applications. Once you get comfortable with Elmโs refreshingly different approach to application development, youโll be working with a clean syntax, dependable libraries, and a delightful compiler that essentially eliminates runtime exceptions. Elm compiles to JavaScript, so your code runs in any browser, and Elmโs best-in-class rendering speed will knock your socks off. Letโs get started!
- 62A Single Page Application written in Elm
https://github.com/rtfeldman/elm-spa-example
A Single Page Application written in Elm. Contribute to rtfeldman/elm-spa-example development by creating an account on GitHub.
- 63dillonkearns/elm-ts-json
https://github.com/dillonkearns/elm-ts-json
Contribute to dillonkearns/elm-ts-json development by creating an account on GitHub.
- 64Sublime Text plugin to run elm-format on save
https://github.com/evancz/elm-format-on-save
Sublime Text plugin to run elm-format on save. Contribute to evancz/elm-format-on-save development by creating an account on GitHub.
- 65A basic UI-clone of Instagram using Elm
https://github.com/bkbooth/Elmstagram
A basic UI-clone of Instagram using Elm. Contribute to bkbooth/Elmstagram development by creating an account on GitHub.
- 66minimum elm + phoenix setup, with webpack
https://github.com/ronanyeah/elm-phoenix-example
minimum elm + phoenix setup, with webpack. Contribute to ronanyeah/elm-phoenix-example development by creating an account on GitHub.
- 67Offline Elm documentation access in your editor
https://github.com/hoelzro/vim-elm-help
Offline Elm documentation access in your editor. Contribute to hoelzro/vim-elm-help development by creating an account on GitHub.
- 68Write Elixir code using statically-typed Elm-like syntax (compatible with Elm tooling)
https://github.com/wende/elchemy
Write Elixir code using statically-typed Elm-like syntax (compatible with Elm tooling) - wende/elchemy
- 69๐ฒ A list of companies using Elm in production.
https://github.com/jah2488/elm-companies
๐ฒ A list of companies using Elm in production. Contribute to jah2488/elm-companies development by creating an account on GitHub.
- 70kalutheo/elm-ui-explorer
https://github.com/kalutheo/elm-ui-explorer
Contribute to kalutheo/elm-ui-explorer development by creating an account on GitHub.
- 71Elm & Guarantees
https://medium.com/@debois/elm-guarantees-92a66679f7bd
In this post, Iโll show you a bug I found in an Elm program recently. This bug is interesting because it shows us both how the guaranteesโฆ
- 72Elm mode for emacs
https://github.com/jcollard/elm-mode
Elm mode for emacs. Contribute to jcollard/elm-mode development by creating an account on GitHub.
- 73Elm project consuming PokรฉAPI
https://github.com/brenopanzolini/pokelmon
Elm project consuming PokรฉAPI. Contribute to brenopanzolini/pokelmon development by creating an account on GitHub.
- 74vendrinc/elm-gql
https://github.com/vendrinc/elm-gql
Contribute to vendrinc/elm-gql development by creating an account on GitHub.
- 75Compiler for Elm, a functional language for reliable webapps.
https://github.com/elm/compiler
Compiler for Elm, a functional language for reliable webapps. - elm/compiler
- 76tinder like app for gifs built with elm and firebase
https://github.com/matthieu-beteille/gipher
tinder like app for gifs built with elm and firebase - matthieu-beteille/gipher
- 77Maintained at: https://github.com/the-sett/elm-serverless
https://github.com/ktonon/elm-serverless
Maintained at: https://github.com/the-sett/elm-serverless - ktonon/elm-serverless
- 78TEA for Bucklescript
https://github.com/OvermindDL1/bucklescript-tea
TEA for Bucklescript. Contribute to OvermindDL1/bucklescript-tea development by creating an account on GitHub.
- 79Elm language plugin for Light Table
https://github.com/rundis/elm-light
Elm language plugin for Light Table. Contribute to rundis/elm-light development by creating an account on GitHub.
- 80put some tailwind in your elm
https://github.com/monty5811/postcss-elm-tailwind
put some tailwind in your elm. Contribute to monty5811/postcss-elm-tailwind development by creating an account on GitHub.
- 81Generates Elm types, JSON decoders, JSON encoders and fuzz tests from JSON schema specifications
https://github.com/dragonwasrobot/json-schema-to-elm
Generates Elm types, JSON decoders, JSON encoders and fuzz tests from JSON schema specifications - dragonwasrobot/json-schema-to-elm
- 82Analyzes Elm projects, to help find mistakes before your users find them.
https://github.com/jfmengels/elm-review
Analyzes Elm projects, to help find mistakes before your users find them. - jfmengels/elm-review
- 83Query for information about values in elm source files.
https://github.com/ElmCast/elm-oracle
Query for information about values in elm source files. - ElmCast/elm-oracle
- 84emmet for vim: http://emmet.io/
https://github.com/mattn/emmet-vim
emmet for vim: http://emmet.io/. Contribute to mattn/emmet-vim development by creating an account on GitHub.
- 85Elm support for Sublime's LSP plugin
https://github.com/sublimelsp/LSP-elm
Elm support for Sublime's LSP plugin. Contribute to sublimelsp/LSP-elm development by creating an account on GitHub.
- 86elm-spa
https://www.elm-spa.dev/
single page apps made easy.
- 87elm-format formats Elm source code according to a standard set of rules based on the official Elm Style Guide
https://github.com/avh4/elm-format
elm-format formats Elm source code according to a standard set of rules based on the official Elm Style Guide - avh4/elm-format
- 88Part One - Getting Started with a New Language
https://www.linkedin.com/pulse/single-page-web-apps-elm-part-one-getting-started-new-kevin-greene
Source on Github: Elm Tutorial Other Posts in this Series Single-Page Web Apps in Elm: Part Two - Functional Routing Single-Page Web Apps in Elm: Part Three - Testing and Structure Single-Page Web Apps in Elm: Part Four - Side Effects Single-Page Web Apps in Elm: Part Five - JavaScript Interoperabil
- 89Elm meets Electron
https://github.com/nirgn975/Elmctron
Elm meets Electron. Contribute to nirgn975/elmctron development by creating an account on GitHub.
- 90Base project for Elm applications
https://github.com/gkubisa/elm-app-boilerplate
Base project for Elm applications. Contribute to gkubisa/elm-app-boilerplate development by creating an account on GitHub.
- 91The friendly community where everyone builds the web
https://glitch.com/search?q=elm&activeFilter=project
Simple, powerful, free tools to create and use millions of apps.
- 92Learn how Elm, Parcel, Cypress and Netlify work together. Get started with Elm navigation, routes, remote data and decoder.
https://github.com/cedricss/elm-batteries
Learn how Elm, Parcel, Cypress and Netlify work together. Get started with Elm navigation, routes, remote data and decoder. - cedricss/elm-batteries
- 93Visualize and edit regular expressions for use in javascript.
https://github.com/johannesvollmer/regex-nodes
Visualize and edit regular expressions for use in javascript. - GitHub - johannesvollmer/regex-nodes: Visualize and edit regular expressions for use in javascript.
- 94Elm and Functional Programming with Richard Feldman (Changelog Interviews #191)
https://changelog.com/podcast/191/
Richard Feldman from NoRedInk joined the show to talk about Elm and Functional Programming. Elm labeled itself "the best of functional programming in your browser" and boasts "no runtime exceptions." We talked about the language, whether or not it's really faster than React, JavaScript fatigue, and the best ways to get...
- 95Elm
https://parceljs.org/languages/elm/
You can import Elm files like any another JavaScript files.
- 96html-to-elm
https://html-to-elm.com/
Turn HTML into Elm. With optional tailwind support.
- 97Elm Radio Podcast
https://elm-radio.com
Tune in to the tools and techniques in the Elm ecosystem.
- 98Elm - The Complete Guide (a web development video tutorial)
https://www.udemy.com/course/elm-the-complete-guide/
Learn the language of the future for web development and increase your productivity and fun using Elm, Elm UI and others
- 99Introduction To The Elm Architecture And How To Build Our First Application | CSS-Tricks
https://css-tricks.com/introduction-elm-architecture-build-first-application/
Creating our first Elm application might seem like a hard task. The new syntax and the new paradigm can be intimidating if you haven't used other functional
- 100Elm - Visual Studio Marketplace
https://marketplace.visualstudio.com/items?itemName=Elmtooling.elm-ls-vscode
Extension for Visual Studio Code - Improving your Elm experience since 2019
- 101Elm Emmet - Visual Studio Marketplace
https://marketplace.visualstudio.com/items?itemName=necinc.elmmet
Extension for Visual Studio Code - Convert emmet abbreviations to composition of elm functions
- 102Autogenerate type-safe GraphQL queries in Elm.
https://github.com/dillonkearns/elm-graphql
Autogenerate type-safe GraphQL queries in Elm. Contribute to dillonkearns/elm-graphql development by creating an account on GitHub.
- 103Elm syntax support for vim
https://github.com/theJian/elm.vim
Elm syntax support for vim. Contribute to theJian/elm.vim development by creating an account on GitHub.
- 104Experiences on the Elm language
https://gist.github.com/ohanhi/0d3d83cf3f0d7bbea9db
Learning FP the hard way: Experiences on the Elm language - frp.md
- 105Elm Camp
https://elm.camp/
An Elm unconference taking place at Colehayes Park, Devon UK. Tue 18th - Fri 21st June
- 106Getting Started with Elm v0.17
https://medium.com/@diamondgfx/getting-started-with-elm-11d7a53b1a78
Last Updated On 05/13/2016
- 107elm-conf 2019
https://2019.elm-conf.com/
elm-conf is a one-day conference for the Elm programming language, returning September 12 2019 to St. Louis, MO.
- 108Elm in the Spring
https://www.youtube.com/channel/UC_wKoNegfKbmVIPg7YYKLWQ
Elm in the Spring is a single-track, single-day conference for developers who love Elm. The first conference took place on Friday, April 26th at the Newberry Library in Chicago.
- 109Elm
https://discourse.elm-lang.org/
Discuss anything Elm related, from beginner questions to compiler design.
- 110In-Depth Elm Tutorials for 2024
https://egghead.io/q?q=elm
Life is too short for long boring videos. Learn Elm using the best screencast tutorial videos online led by working professionals that learn in public.
- 111SwiftUI Overview - Xcode - Apple Developer
https://developer.apple.com/xcode/swiftui/
SwiftUI is an innovative, exceptionally simple way to build user interfaces across all Apple platforms with the power of Swift.
- 112Welcome to Elm!
https://www.youtube.com/playlist?list=PLuGpJqnV9DXq_ItwwUoJOGk_uCr72Yvzb
A complete Elm tutorial designed for folks coming from HTML/CSS/JS
- 113From Authentication to Calling an API (Part 1)
https://auth0.com/blog/creating-your-first-elm-app-part-1/
Explore building an app in the functional front-end language Elm. Part 1 focuses on Elm history, architecture, syntax, and calling an API...
- 114Elm and Functional Programming with Evan Czaplicki and Richard Feldman (Changelog Interviews #218)
https://changelog.com/podcast/218
Evan Czaplicki, creator of Elm, and Richard Feldman of NoRedInk joined the show to talk deeper about Elm, the pains of CSS it solves, scaling the Elm architecture, reusable components, and more.
- 115Tour of an Open-Source Elm SPA
https://dev.to/rtfeldman/tour-of-an-open-source-elm-spa
How to organize an Elm single page application.
- 116Introducing .NET Multi-platform App UI - .NET Blog
https://devblogs.microsoft.com/dotnet/introducing-net-multi-platform-app-ui/
You can build anything with .NET. Itโs one of the main reasons millions of developers choose .NET as the platform for their careers, and companies invest for their businesses. With .NET 5 we begin our journey of unifying the .NET platform, bringing .NET Core and Mono/Xamarin together in one base class library (BCL) and toolchain [โฆ]
- 117Evan Czaplicki - Let's be mainstream! User focused design in Elm - Curry On
https://www.youtube.com/watch?v=oYk8CKH7OhE
Curry On Prague, July 7th 2015http://curry-on.orghttp://2015.ecoop.org
- 118OpenAPI Generator allows generation of API client libraries (SDK generation), server stubs, documentation and configuration automatically given an OpenAPI Spec (v2, v3)
https://github.com/OpenAPITools/openapi-generator
OpenAPI Generator allows generation of API client libraries (SDK generation), server stubs, documentation and configuration automatically given an OpenAPI Spec (v2, v3) - OpenAPITools/openapi-gener...
- 119Effects as Data | Richard Feldman | Reactive 2015
https://www.youtube.com/watch?v=6EdXaWfoslc
Imagine a world without side effects, where the only way to make things happen was to call functions whose return values described what you wanted done. What...
- 120Amitai Burstein - Elm - Frontend with Guarantees
https://www.youtube.com/watch?v=FgaoOgJ5CAU
You had enough of Angular, and as good as React may be, you are still trying to figure out what router library to use and how to setup your project. In short...
- 121Build a Web App Using Functional Programming Principles with Elm
https://www.sitepoint.com/premium/courses/elm-a-beginners-guide-to-elm-and-data-2940
Build a Web App Using Functional Programming Principles with Elm - Elm, a front-end functional programming language for web apps
- 122Building Applications in Elm
https://www.youtube.com/watch?v=txxKx_I39a8
React re-thought best practices for web and native development, but it is limited by the strengths and weaknesses of JavaScript.Elm takes rethinking best pra...
- 123"Making Impossible States Impossible" by Richard Feldman
https://www.youtube.com/watch?v=IcgmSRJHu_8
Among the most time-consuming bugs to track down are the ones where we look at our application state and say "this shouldnโt be possible."We can use Elmโs co...
- 124Generate Elm types, encoders, and decoders from Haskell types
https://github.com/folq/haskell-to-elm
Generate Elm types, encoders, and decoders from Haskell types - haskell-to-elm/haskell-to-elm
- 125Adventures in Elm โข Jessica Kerr โข GOTO 2016
https://www.youtube.com/watch?v=cgXhMc8M4X4
This presentation was recorded at GOTO Chicago 2016. #gotocon #gotochgohttp://gotochgo.comJessica Kerr - Polyglot Functional Developer @jessitronica ABSTRACT...
- 126Elm & Components
https://medium.com/p/elm-components-3d9c00c6c612
The Elm Architecture (TEA) is conceptually very nice, but it forces us to write large amounts of boilerplate whenever we need to use aโฆ
- 127A nice app on Elm street
https://madewithlove.com/blog/software-engineering/using-elm-with-react-a-nice-app-on-elm-street/
A complete guide on how to use Elm with React: If you've ever worked with Redux โ in the context of a React application or not โ you may have heard numerous times that it was inspired not only by Flux (which it followed) but also by the Elm architecture.
- 128Boilerplate for developing Elm apps on Webpack
https://github.com/moarwick/elm-webpack-starter
Boilerplate for developing Elm apps on Webpack. Contribute to elm-community/elm-webpack-starter development by creating an account on GitHub.
- 129F# | Succinct, robust and performant language for .NET
https://dotnet.microsoft.com/languages/fsharp
F# is an open-source, cross-platform, functional programming language for the .NET developer platform. Develop with free tools for Linux, macOS, and Windows.
- 130Writing Native
https://github.com/NoRedInk/take-home/wiki/Writing-Native
A take-home application server written in Elm and only Elm - eeue56/take-home
- 131Creating an Elm App with IHP
https://www.youtube.com/watch?v=b9ULHutH6ag
Continue here: https://driftercode.com/blog/ihp-with-elm-series
- 132An Elm plugin for protoc
https://github.com/andreasewering/protoc-gen-elm
An Elm plugin for protoc. Contribute to anmolitor/protoc-gen-elm development by creating an account on GitHub.
- 133A showcase of awesome programming language projects and resources written in Elm.
https://github.com/pd-andy/awesome-elm-pltd
A showcase of awesome programming language projects and resources written in Elm. - hayleigh-dot-dev/awesome-elm-pltd
- 134๐ฆ Very very basic elm + webpack 4 template
https://github.com/FranzSkuffka/elm-webpack-starter-kid
๐ฆ Very very basic elm + webpack 4 template. Contribute to janwirth/elm-webpack-starter-kid development by creating an account on GitHub.
- 135Compile time internationalization for Elm supporting multiple input and output formats
https://github.com/andreasewering/travelm-agency
Compile time internationalization for Elm supporting multiple input and output formats - anmolitor/travelm-agency
- 136Elm plugin for IntelliJ Platform IDEs
https://github.com/klazuka/intellij-elm
Elm plugin for IntelliJ Platform IDEs. Contribute to intellij-elm/intellij-elm development by creating an account on GitHub.
- 137Features โข Security
https://dependabot.com
Build on a secure foundation.
- 138Elm Basics
https://www.youtube.com/watch?v=g48K6ABfRzA
Andy Balaam takes you through all the syntax and basic ideas in Elm as a general programming language, not covering the Elm Architecture, but just how to rea...
- 139Elm 0.19 webpack 4 starter template to build SPA
https://github.com/romariolopezc/elm-webpack-4-starter
Elm 0.19 webpack 4 starter template to build SPA. Contribute to rlopzc/elm-webpack-starter development by creating an account on GitHub.
- 140Elm from a Business Perspective
http://www.gizra.com/content/elm-business-perspective/
Elm is not just technically great, it also allows building business around it
- 141denisorehovsky/django-elm-auth-with-jwt
https://github.com/apirobot/django-elm-auth-with-jwt
Contribute to denisorehovsky/django-elm-auth-with-jwt development by creating an account on GitHub.
- 142Electronic Cookbook
https://github.com/theiceshelf/arisgarden
Electronic Cookbook. Contribute to teesloane/arisgarden development by creating an account on GitHub.
- 143Elm Weekly | Wolfgang Schuster | Substack
http://www.elmweekly.nl/
Bringing you the best Elm content to your inbox since 2016. Click to read Elm Weekly, by Wolfgang Schuster, a Substack publication with thousands of subscribers.
- 144Prior Art | Redux
https://redux.js.org/introduction/prior-art
Understanding > Prior Art: Influences on the design of Redux
- 145A cross-platform GUI library for Rust, inspired by Elm
https://github.com/hecrj/iced
A cross-platform GUI library for Rust, inspired by Elm - iced-rs/iced
- 146Generate types and converters from JSON, Schema, and GraphQL
https://github.com/quicktype/quicktype
Generate types and converters from JSON, Schema, and GraphQL - glideapps/quicktype
- 147Declarative UI framework for cross-platform mobile & desktop apps, using MVU and F# functional programming
https://github.com/fsprojects/Fabulous
Declarative UI framework for cross-platform mobile & desktop apps, using MVU and F# functional programming - fabulous-dev/Fabulous
- 148elm-pages - pull in typed elm data to your pages
http://elm-pages.com
pull in typed elm data to your pages
- 149Live reloading server for Elm development
https://elm-live.com/
Documentation and more for elm-live, a dev server for elm development with live reloading.
- 150Sunsetting Atom
https://atom.io/packages/elm-instant
We are archiving Atom and all projects under the Atom organization for an official sunset on December 15, 2022.
- 151Richard Feldman Discusses Elm and How It Compares to React.js for Front-End Programming
https://www.infoq.com/podcasts/richard-feldman
Richard Feldman talks about Elm, a front-end focused functional programming language that compiles to JavaScript. Feldman covers being an early adopter of Elm, the architecture of Elm, immutability, semantic versioning and more. He also compares Elm to some popular JavaScript frameworks including React.
- 152Sunsetting Atom
https://atom.io/packages/language-elm
We are archiving Atom and all projects under the Atom organization for an official sunset on December 15, 2022.
- 153Sunsetting Atom
https://atom.io/packages/elm-navigator
We are archiving Atom and all projects under the Atom organization for an official sunset on December 15, 2022.
- 154Sunsetting Atom
https://atom.io/packages/linter-elm-make
We are archiving Atom and all projects under the Atom organization for an official sunset on December 15, 2022.
- 155Sunsetting Atom
https://atom.io/packages/elmjutsu
We are archiving Atom and all projects under the Atom organization for an official sunset on December 15, 2022.
- 156Elm in the Spring 2020
https://www.elminthespring.org/
A one-day, single-track Elm conference. Join us for a great day of learning, teaching, and community!
- 157Elm with Richard Feldman and Srinivas Rao - Software Engineering Daily
http://softwareengineeringdaily.com/2015/11/03/elm-with-richard-feldman-and-srinivas-rao/
"There are entire days where I donโt even look at the browser when Iโm coding in Elm because I just know its going to work.โ Elm is a functional programming language for web browsers. Continue readingโฆ
- 158Richard Feldman - Exploring elm-spa-example
https://youtu.be/RN2_NchjrJQ
It's been almost 2 years since the widely referenced elm-spa-example's release in 2017, and a lot has changed! Where did all those new techniques come from? ...
- 159Joel Clermont - What the heck is Elm?
http://www.fullstackradio.com/44
In this episode, Adam talks to Joel Clermont about the Elm programming language and getting started with functional programming.
- 160Elm Tutorials and Insights | Codementor Community
https://www.codementor.io/elm/tutorial
Learn about the latest trends in Elm. Read tutorials, posts, and insights from top Elm experts and developers for free.
- 161Elm in Production" by Richard Feldman
http://www.youtube.com/watch?v=FV0DXNB94NE
How often do you find a back-end team jealous of the language the front-end team gets to use? Having modernized many legacy front-ends over the years, I can ...
- 162Elm on Exercism
http://exercism.io/languages/elm
Get fluent in Elm by solving 93 exercises. And then level up with mentoring from our world-class team.
- 163How elm programming language made our world better
http://futurice.com/blog/elm-in-the-real-world
Elm is a beginner friendly functional reactive programming language for building web frontend. Choosing Elm for a customer project made my job nicer than ever and helped maintain project velocity during months of development.
Related Articlesto learn about angular.
- 1Why Learn Elm? Guide to Functional Front-End Development
- 2Functional Programming Basics in Elm: Immutability, Pure Functions, and Recursion
- 3Building Your First Web App with Elm
- 4Building Forms and Handling User Input in Elm
- 5How Elm Manages State and Updates with Elm Architecture
- 6Advanced Patterns in the Elm Architecture: Decoders, Commands, and Subscriptions
- 7Optimizing Performance in Large Elm Applications
- 8Debugging and Testing in Elm: Robust and Error-Free Applications
- 9Interoperability: Elm with JavaScript for Real-World Applications
- 10Building an Elm and GraphQL API-Powered Web Application
FAQ'sto learn more about Angular JS.
mail [email protected] to add more queries here ๐.
- 1
what resources are available for learning Elm
- 2
what are the performance considerations when using Elm for complex applications
- 3
how does Elm fit into a modern DevOps pipeline
- 5
how does Elm handle asynchronous operations and side effects
- 7
what role does Elm play in the broader context of functional programming languages
- 8
what challenges do developers face when using Elm
- 9
what are the benefits of using Elm's architecture for managing application state
- 10
what are the advantages of using Elm over JavaScript
- 11
how does Elm's functional programming paradigm impact development
- 12
is Elm programming language dead
- 13
what is Elm software
- 14
will Elm be popular
- 15
how does Elm's compiler contribute to a better developer experience
- 16
is Elm a good choice for new web projects
- 17
how can Elm be integrated with other technologies or languages
- 18
how does Elm compare to other front-end frameworks
- 19
what kind of applications are best suited for Elm development
- 20
what are the latest updates in Elm programming
- 21
what are some common misconceptions about Elm
- 22
can Elm be used for server-side development
- 23
what are the best practices for using Elm in large-scale applications
- 24
do electrical engineers program in Elm
- 25
is there a growing demand for Elm developers
- 26
is Elm still being developed
- 27
what are the key features of the Elm programming language
- 28
are there major companies using Elm for their projects
- 29
why is Elm programming important
- 31
are there any major open-source libraries or tools for Elm
- 32
who created Elm programming
- 33
how does Elm's approach to immutability differ from other languages
- 34
will Elm be used to make websites
- 35
how active is the Elm development community
- 36
what is Elm programming
- 37
how can developers contribute to the Elm ecosystem
- 38
what is Elm language
- 39
is Elm language dead
- 40
how does Elm's type system benefit web development
- 41
do electrical engineers learn Elm programming
- 42
who programmed elm programming
- 43
how does Elm handle state management compared to other frameworks
- 44
what are some notable Elm projects or success stories
- 45
how does Elm's performance compare to other front-end technologies
- 46
what are the most common use cases for Elm in industry
- 47
what is Elm programming language
- 48
are there any notable Elm conferences or community events
- 49
what is the future of Elm in the programming landscape
- 50
does Elm work in modern time
- 51
what are the challenges of transitioning to Elm from JavaScript
More Sitesto check out once you're finished browsing here.
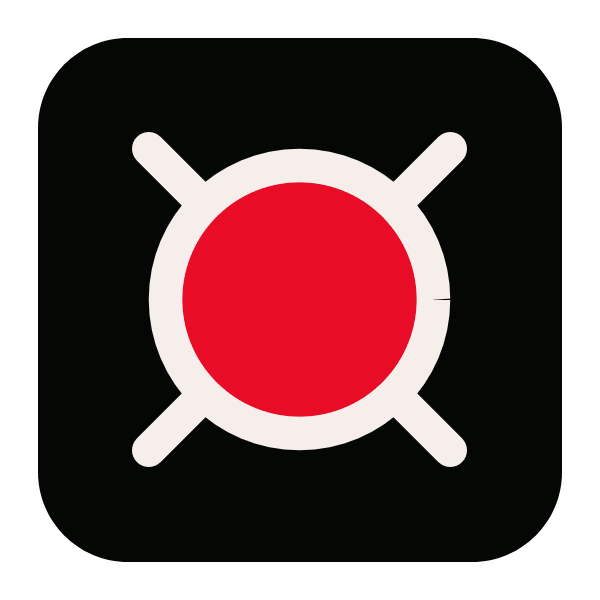
https://www.0x3d.site/
0x3d is designed for aggregating information.
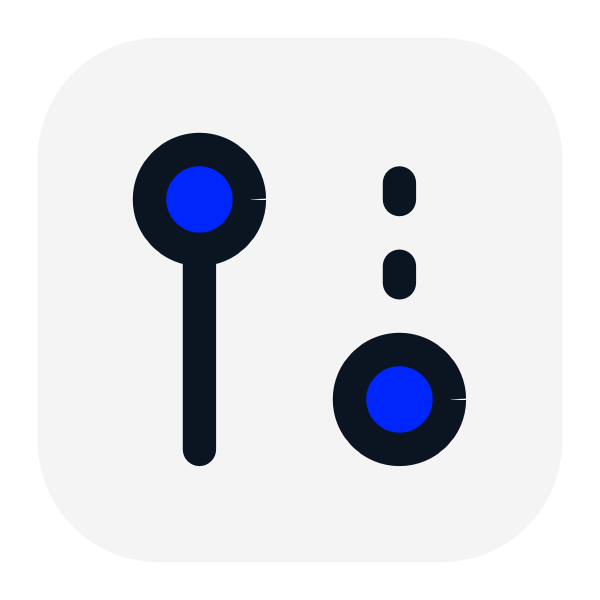
https://nodejs.0x3d.site/
NodeJS Online Directory

https://cross-platform.0x3d.site/
Cross Platform Online Directory
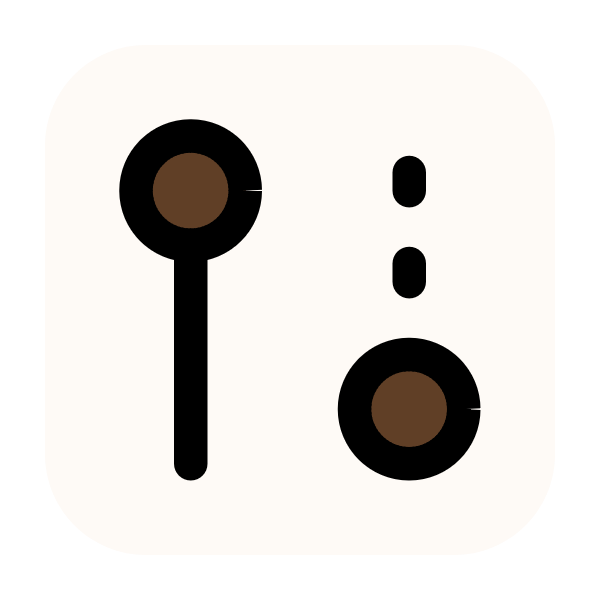
https://open-source.0x3d.site/
Open Source Online Directory
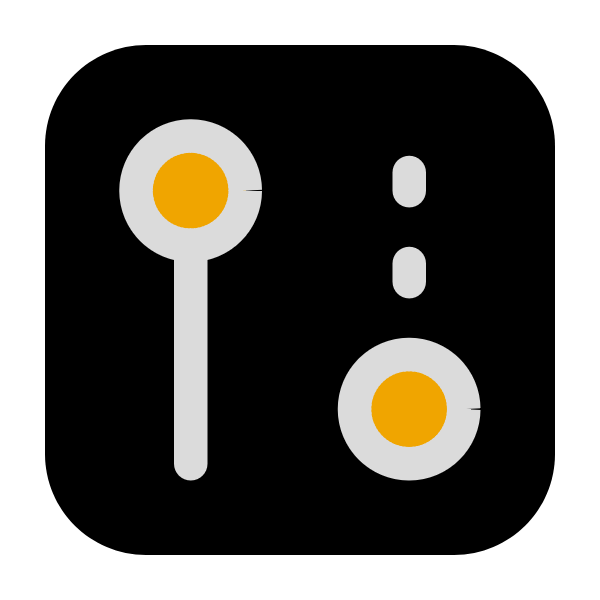
https://analytics.0x3d.site/
Analytics Online Directory
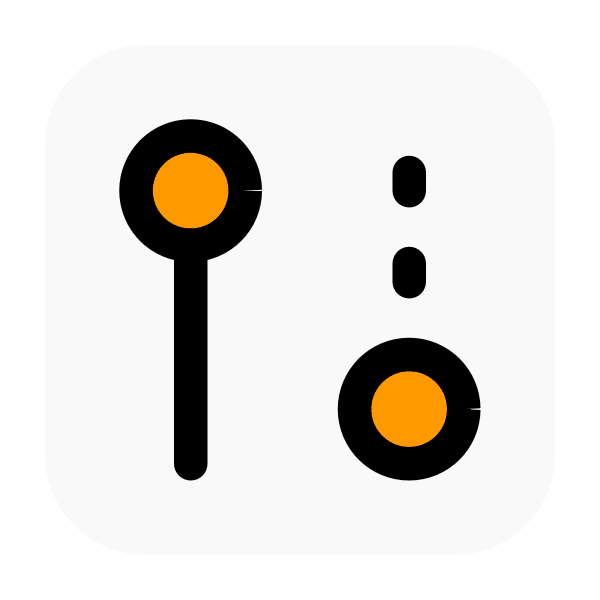
https://javascript.0x3d.site/
JavaScript Online Directory

https://golang.0x3d.site/
GoLang Online Directory
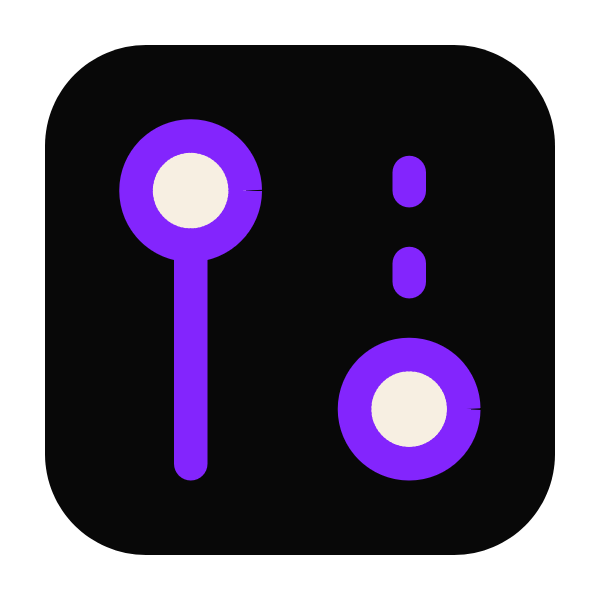
https://python.0x3d.site/
Python Online Directory

https://swift.0x3d.site/
Swift Online Directory

https://rust.0x3d.site/
Rust Online Directory
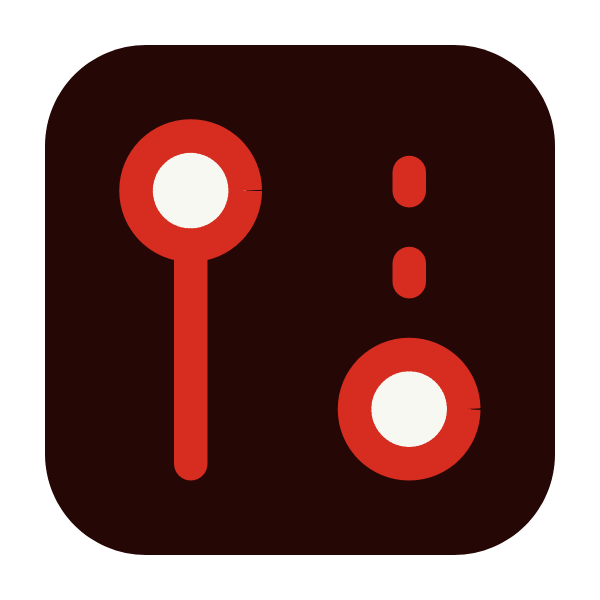
https://scala.0x3d.site/
Scala Online Directory

https://ruby.0x3d.site/
Ruby Online Directory

https://clojure.0x3d.site/
Clojure Online Directory
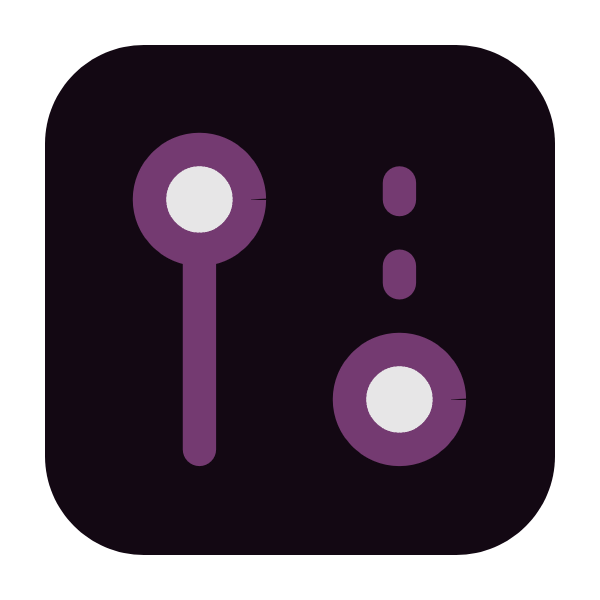
https://elixir.0x3d.site/
Elixir Online Directory
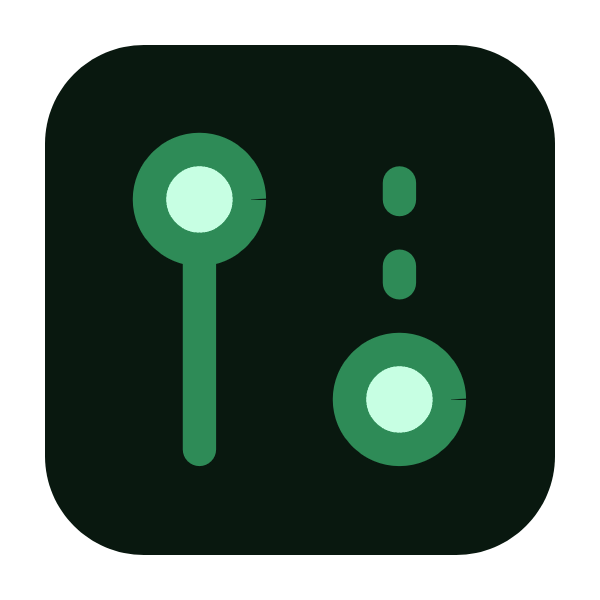
https://elm.0x3d.site/
Elm Online Directory

https://lua.0x3d.site/
Lua Online Directory
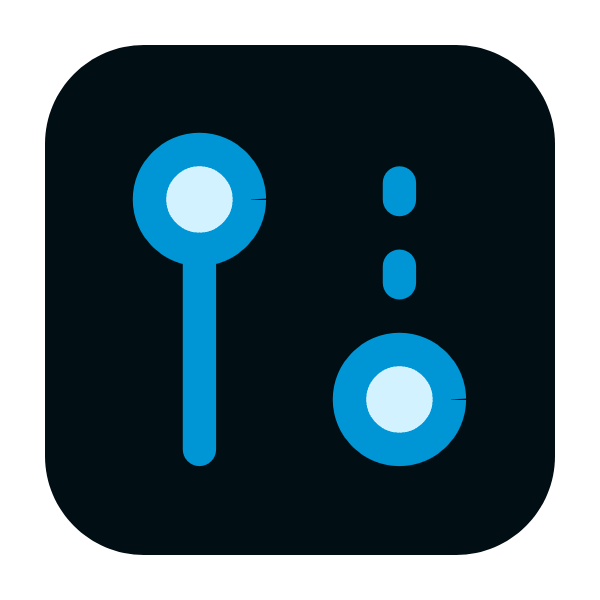
https://c-programming.0x3d.site/
C Programming Online Directory
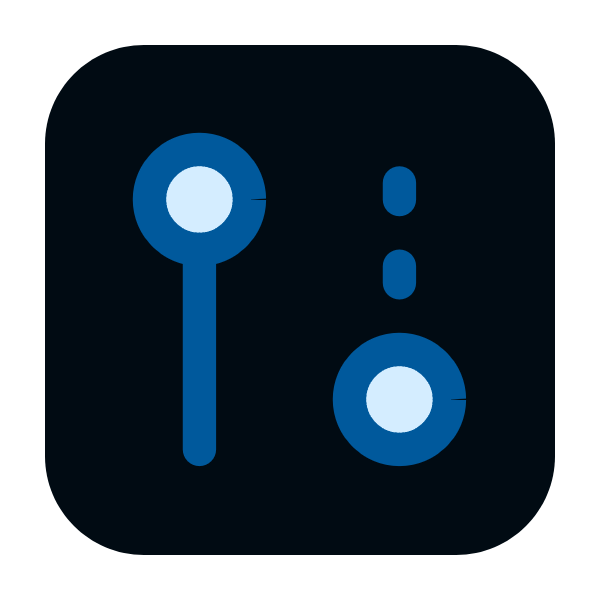
https://cpp-programming.0x3d.site/
C++ Programming Online Directory

https://r-programming.0x3d.site/
R Programming Online Directory

https://perl.0x3d.site/
Perl Online Directory
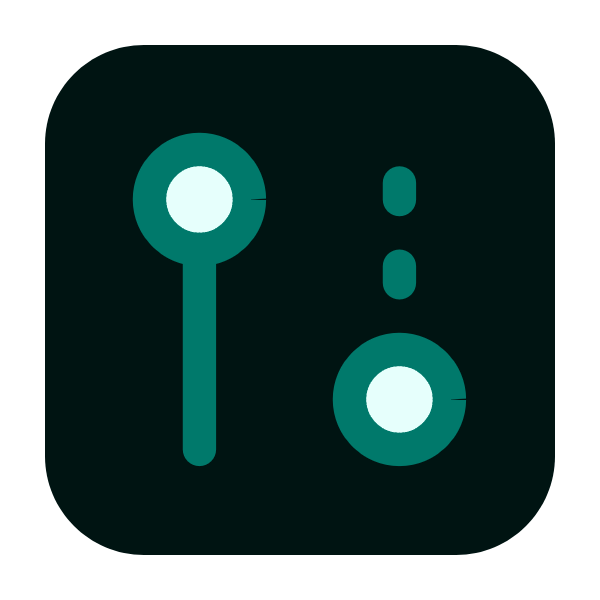
https://java.0x3d.site/
Java Online Directory

https://kotlin.0x3d.site/
Kotlin Online Directory

https://php.0x3d.site/
PHP Online Directory

https://react.0x3d.site/
React JS Online Directory
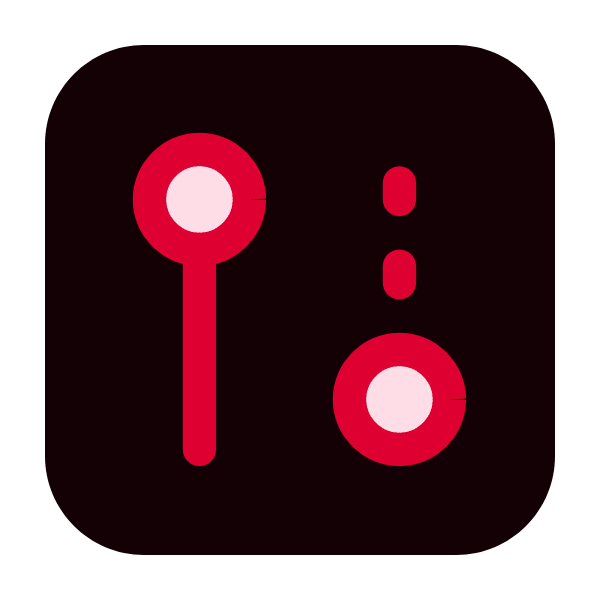
https://angular.0x3d.site/
Angular JS Online Directory